0
0
0
share
#laravel#php#Tutorial#web-framework
0 Reactions
0 Komentar
Memulai Web Development dengan Laravel - Menjajal Controller
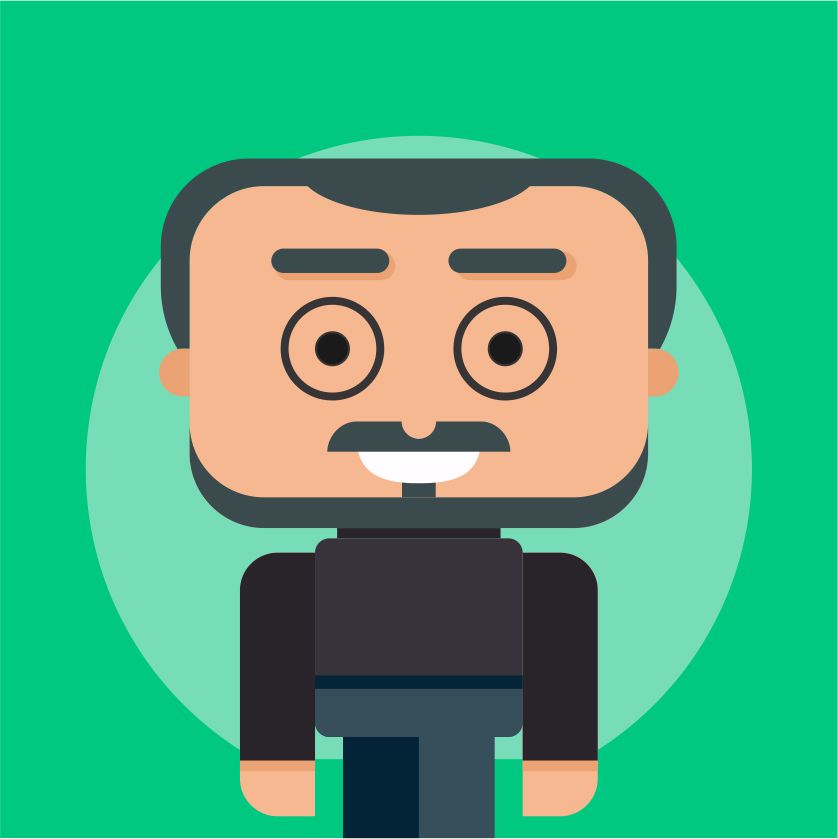
Muhammad Arslan • 14 April 2017
.jpg)
Controller adalah sebuah class yang digunakan untuk menangani request dari client dan mengarahkannya ke view tertentu atau menjalankan suatu proses bisnis yang disimpan di model. Di dalamnya dapat juga melakukan trigger terhadap notifikasi atau jobs yang akan dieksekusi di kemudian waktu.
Controller di Laravel diharuskan untuk meng-extend dari class App\Http\Controllers\Controller. Untuk penamaan, disarankan untuk menggunakan title di setiap nama controller dan nama controller diakhiri dengan kata Controller.
Setiap method di dalamnya, dapat dipanggil untuk dikaitkan dengan route yang akan menentukan method mana di suatu controller yang akan menangani request dari URL tertentu. Sekarang kita akan mencoba mengenal beberapa cara membuat Controller di Laravel.
Controller di Laravel
Sekarang silahkan buat file di dalam folder app/Http/Controllers kemudian beri nama dengan HelloController. Berikut adalah contoh kode controller untuk HelloController:
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
class HelloController extends Controller {
public function index() {
echo "<h1>Hello world!</h1>";
}
public function showPostComments($id) {
echo "Sedang menampilkan komentar pada postingan dengan id: <b>".$id."</b>";
}
public function showPosts($id=0) {
echo "Sedang menampilkan postingan dengan id: <b>".$id."</b>";
}
public function showPostArchive($year, $month, $day) {
echo "Sedang menampilkan postingan pada tanggal ".$year."-".$month."-".$day;
}
public function testRedirect() {
return redirect("hello");
}
}
Seperti yang kamu lihat, suatu method di Controller dapat memiliki satu atau banyak parameter, juga dapat diberi nilai default bila tidak diisi saat mengakses URL. Untuk penamaan diharuskan camel case.
Untuk redirect, kamu dapat menggunakan function bawaan Laravel yaitu redirect() dengan melewatkan URL yang ingin dituju.
Pastikan kamu mengikuti best practice Laravel agar web developer lain yang sudah menggunakan Laravel dapat bergabung dengan mudah di proyek yang kamu bangun.
Basic Route
Untuk menempelkan controller ke Laravel, kamu harus mendefinisikannya di dalam routes/web.php. Di Laravel ada beberapa method untuk menentukan suatu route berdasarkan HTTP Verb.
- Route::get()
- Route::post()
- Route::put()
- Route::delete()
- Route::patch()
- Route::options()
Ada juga beberapa method khusus seperti Route::match(), Route::any(), dan Route::resource().
Silahkan tambahkan route untuk HelloController seperti pada kode berikut:
<?php
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| This file is where you may define all of the routes that are handled
| by your application. Just tell Laravel the URIs it should respond
| to using a Closure or controller method. Build something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
// contoh dasar
Route::get('/hello/', 'HelloController@index');
Route::get('/hello/posts/{id}', 'HelloController@showPosts');
Route::get('/hello/posts/{id}/comment', 'HelloController@showPostComments');
Route::get('/hello/posts/{year}/{month}/{day}', 'HelloController@showPostArchive');
Route::get('/hello/test_redirect', 'HelloController@testRedirect');
Bila tidak ada masalah, berikut adalah contoh URL yang dapat diakses di browser untuk HelloController:
http://localhost/laraweb/public/index.php/hello
http://localhost/laraweb/public/index.php/hello/posts/1
http://localhost/laraweb/public/index.php/hello/posts/1/comment
http://localhost/laraweb/public/index.php/hello/posts/2016/07/23
http://localhost/laraweb/public/index.php/hello/test_redirect
Grouped Route
Sekarang kita akan mencoba hal yang sedikit berbeda, dimana kita akan mencoba membuat sebuah controller yang berada di dalam sebuah direktori. Silahkan buat sebuah direktori dengan nama Media di dalam app/Http/Controllers. Kemudian buatlah AudioController.php di dalam file tersebut dan buatlah kode berikut di dalam file tersebut:
<?php
namespace App\Http\Controllers\Media;
use App\Http\Controllers\Controller;
class AudioController extends Controller {
public function index() {
echo "Sedang menampilkan daftar audio...";
}
public function show($id) {
echo "Sedang menampilkan audio dengan id: <b>".$id."</b>";
}
public function create() {
echo "Sedang mengunggah file audio baru";
}
public function delete($id) {
echo "Sedang menghapus file audio dengan id: <b>".$id."</b>";
}
}
Dan di dalam routes/web.php, akan kita tambahkan grouped route untuk AudioController yang digrup berdasarkan namespace. Disini kita gunakan "namespace"=>"Media" karena file AudioController berada di dalam folder Media dan namespace-nya adalah App\Http\Controllers\Media:
// contoh tanpa grouped
/*
Route::get("/audio/", "Media\AudioController@index");
Route::get("/audio/new", "Media\AudioController@create");
Route::get("/audio/{id}", "Media\AudioController@show");
Route::get("/audio/{id}/delete", "Media\AudioController@delete");
*/
// contoh grouped
Route::group(["namespace"=>"Media"], function(){
Route::get("/audio/", "AudioController@index");
Route::get("/audio/new", "AudioController@create");
Route::get("/audio/{id}", "AudioController@show");
Route::get("/audio/{id}/delete", "AudioController@delete");
});
Berikut adalah URL yang dapat diakses di browser untuk AudioController:
http://localhost/laraweb/public/index.php/audio
http://localhost/laraweb/public/index.php/audio/new
http://localhost/laraweb/public/index.php/audio/119
http://localhost/laraweb/public/index.php/audio/119/delete
Resource Controller
Resource Controller adalah sebutan untuk controller yang mengikuti kaidah HTTP Verb pada RESTful API. Namun sejatinya itu hanyalah template yang sudah diberi nama standard yang akan dimuat dengan menggunakan Route::resource(). Method yang ada di dalam Resource Controller antara lain:
- index, menampilkan daftar item
- show, menampilkan suatu item
- create, menampilkan form tambah
- store, menambahkan item baru
- edit, menampilkan form untuk mengedit salah satu item
- update, mengubah suatu item
- destroy, menghapus suatu item
Method diatas akan dimuat secara otomatis bila menggunakan Route::resource(). Untuk membuat resource controller, kita dapat menggunakan artisan command seperti berikut:
$ php artisan make:controller PageController --resource
$ php artisan make:controller FlightController --resource
$ php artisan make:controller HotelController --resource
Controller hasil generate dengan artisan command tadi akan disimpan di dalam folder app\Http\Controllers.
Berikut adalah isi file PageController.php yang harus kamu ikuti:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PageController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: index page";
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
echo "GET: create page";
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
echo "POST: store page";
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
echo "GET: show page";
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
echo "GET: edit page";
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
echo "GET: update page";
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
echo "DEL: destroy page";
}
}
Dan berikut adalah isi file FlightController.php yang sudah di-edit (silahkan buat kode berikut di dalam FlightController.php):
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FlightController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: flight index";
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
echo "GET: flight show";
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
Begitu juga dengan file HotelController.php, silahkan edit beberapa bagian sesuai dengan kode dibawah ini:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HotelController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: hotel index";
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
echo "GET: hotel create";
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
echo "GET: hotel show";
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
Dan sekarang silakhan tambahkan route untuk ketiga controller tadi di dalam routes/web.php:
// contoh resource
Route::resource('page', "PageController");
Route::resource('flight', "FlightController", ["only" =>
["index", "show"]
]);
Route::resource('hotel', "HotelController", ["except" =>
["store", "edit", "update", "destroy"]
]);
Pada kode diatas, ada sedikit perbedaan dalam penggunaan Route::resource(). Untuk PageController, kita muat semuat methodnya. Sedangkan untuk FlightController kita hanya membuat index dan show. Lalu untuk HotelController hanya kita muat selain store, edit, update, dan destroy.
Berikut adalah URL yang dapat diakses di browser untuk ketiga controller diatas:
GET http://localhost/laraweb/public/index.php/page
POST http://localhost/laraweb/public/index.php/page/create
POST http://localhost/laraweb/public/index.php/page
GET http://localhost/laraweb/public/index.php/page/1
GET http://localhost/laraweb/public/index.php/page/1/edit
PUT http://localhost/laraweb/public/index.php/page/1
DELETE http://localhost/laraweb/public/index.php/page/1
GET http://localhost/laraweb/public/index.php/flight
GET http://localhost/laraweb/public/index.php/flight/1
GET http://localhost/laraweb/public/index.php/hotel
GET http://localhost/laraweb/public/index.php/hotel/1
GET http://localhost/laraweb/public/index.php/hotel/create
Route Prefix
Sekarang kita akan mencoba hal yang sama seperti grouped route hanya saja kita akan menambahkan prefix di URL yang seharusnya. Silahkan buat dulu folder Admin di dalam app\Http\Controllers.
Silahkan buat tiga controller berikut dengan menggunakan artisan command:
$ php artisan make:controller Admin\\UsersController --resource
$ php artisan make:controller Admin\\CategoriesController --resource
$ php artisan make:controller Admin\\PostsController --resource
Silahkan sesuaikan kodenya pada file Admin/UsersController.php dengan kode berikut ini:
<?php
namespace App\Http\Controllers\Admin;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class UsersController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: admin/users/index";
}
.......................................
Silahkan sesuaikan kodenya pada file Admin/CategoriesController.php dengan kode berikut ini:
<?php
namespace App\Http\Controllers\Admin;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class CategoriesController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: admin/categories/index";
}
.......................................
Silahkan sesuaikan kodenya pada file Admin/PostsController.php dengan kode berikut ini:
<?php
namespace App\Http\Controllers\Admin;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class PostsController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
echo "GET: admin/posts/index";
}
.......................................
Lalu tambahkan route untuk ketiga controller tadi di dalam routes/web.php:
// contoh route prefix
Route::group(["namespace"=>"Admin", "prefix"=>"admin"], function(){
Route::resource('users', "UsersController", ["only" =>
["index"]
]);
Route::resource('posts', "PostsController", ["only" =>
["index"]
]);
Route::resource('categories', "CategoriesController", ["only" =>
["index"]
]);
});
Hasilnya berikut adalah URL yang dapat diakses di browser untuk ketiga controller tadi:
http://localhost/laraweb/public/index.php/admin/users
http://localhost/laraweb/public/index.php/admin/categories
http://localhost/laraweb/public/index.php/admin/posts