Belajar Membuat REST API Sederhana dengan Django dan Tastypie
Bagus Aji Santoso 7 Desember 2017
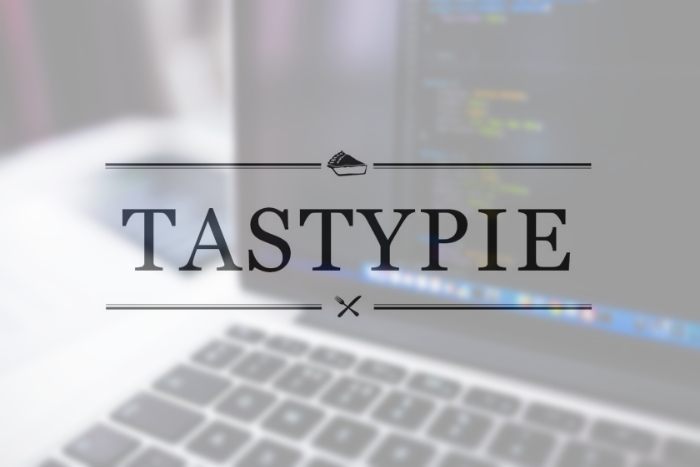
Persiapan
Tutorial ini menggunakan Python (v3.5.1), Django (v1.9.7), dan django-tastypie(v13.3)
Buat sebuah direktori project baru:
$ mkdir django-tastypie-tutorial
$ cd django-tastypie-tutorial
Kemudian buat sebuah virtualenv dan aktifkan:
$ pyvenv-3.5 env
$ source env/bin/activate
REST API Server Sederhana dengan Codeigniter 3
Setelah virtualenv aktif, pasang dependensi yang diperlukan:
$ pip install Django==1.9.7
$ pip install django-tastypie==0.13.3
$ pip install defusedxml==0.4.1
$ pip install lxml==3.6.0
Selanjutnya buat sebuah project Django dan sebuah app bernama whatever
:
$ django-admin.py startproject django19
$ cd django19
$ python manage.py startapp whatever
Pastikan untuk menambahkan app yang baru dibuat ke INSTALLED_APPS
di settings.py:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'whatever',
]
Kita akan menggunakan database SQLite, pastikan sudah ada pengaturan berikut di settings.py (pembaca bisa menggunakan database yang lain):
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'test.db'),
}
}
Lalu update file models.py dengan menambahkan sebuah kelas data:
from django.db import models
class Whatever(models.Model):
title = models.CharField(max_length=200)
body = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
Buat dan lakukan migrasi database:
$ python manage.py makemigrations
$ python manage.py migrate --fake-initial
Catatan: fake-initial
adalah argumen tambahan jika kita ingin memeriksa apakah sudah ada tabel dengan nama yang sama. Jika ada, migrasi dibatalkan.
Buka Django Shell untuk mengisi beberapa data:
$ python manage.py shell
>>> from whatever.models import Whatever
>>> w = Whatever(title="What Am I Good At?", body="What am I good at? What is my talent? What makes me stand out? These are the questions we ask ourselves over and over again and somehow can not seem to come up with the perfect answer. This is because we are blinded, we are blinded by our own bias on who we are and what we should be. But discovering the answers to these questions is crucial in branding yourself.")
>>> w.save()
>>>
>>> w = Whatever(title="Charting Best Practices: Proper Data Visualization", body="Charting data and determining business progress is an important part of measuring success. From recording financial statistics to webpage visitor tracking, finding the best practices for charting your data is vastly important for your company’s success. Here is a look at five charting best practices for optimal data visualization and analysis.")
>>> w.save()
>>>
>>> w = Whatever(title="Understand Your Support System Better With Sentiment Analysis", body="There’s more to evaluating success than monitoring your bottom line. While analyzing your support system on a macro level helps to ensure your costs are going down and earnings are rising, taking a micro approach to your business gives you a thorough appreciation of your business’ performance. Sentiment analysis helps you to clearly see whether your business practices are leading to higher customer satisfaction, or if you’re on the verge of running clients away.")
>>> w.save()
Keluar dari shell setelah selesai dengan CTRL+D atau perintah exit.
Pengaturan Tastypie
Buat sebuah file dengan nama api.py:
from tastypie.resources import ModelResource
from tastypie.constants import ALL
from whatever.models import Whatever
class WhateverResource(ModelResource):
class Meta:
queryset = Whatever.objects.all()
resource_name = 'whatever'
filtering = {'title': ALL}
File ini lah yang bertugas membaca database lalu mengembalikannya dalam bentuk yang diinginkan.
Perbarui urls.py untuk menambahkan routing:
from django.conf.urls import url, include
from django.contrib import admin
from django19.api import WhateverResource
whatever_resource = WhateverResource()
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^api/', include(whatever_resource.urls)),
]
Testing
Coba jalankan server Django dengan:
$ python manage.py runserver
Akses http://localhost:8000/api/whatever/?format=json untuk mengambil data dalam format JSON.
Akses http://localhost:8000/api/whatever/?format=xml untuk mengambil data dalam format XML.
Masih ingat dengan baris kode berikut di kelas WhateverResource
?
filtering = {'title': ALL}
Karena baris tersebut, kita bisa melakukan filter berdasarkan title
(properti kelas Whatever
). Contoh:
http://localhost:8000/api/whatever/?format=json&title__contains=what
http://localhost:8000/api/whatever/?format=json&title__contains=test
Membuat REST API Sederhana Dengan Django
Penutup
Tastypie memiliki kemampuan yang jauh lebih lengkap dari yang kita bahas di artikel ini. Kunjungi situs resminya disini untuk melihat informasi lebih lanjut seputar Tastypie.
Kode tutorial ini dapat diakses di github.
Diterjemahkan dari Create a Super Basic REST API With Django Tastypie dipublikasikan oleh Real Python