Membuat Helper Sendiri di Laravel
Anugrah Sandi 10 November 2017
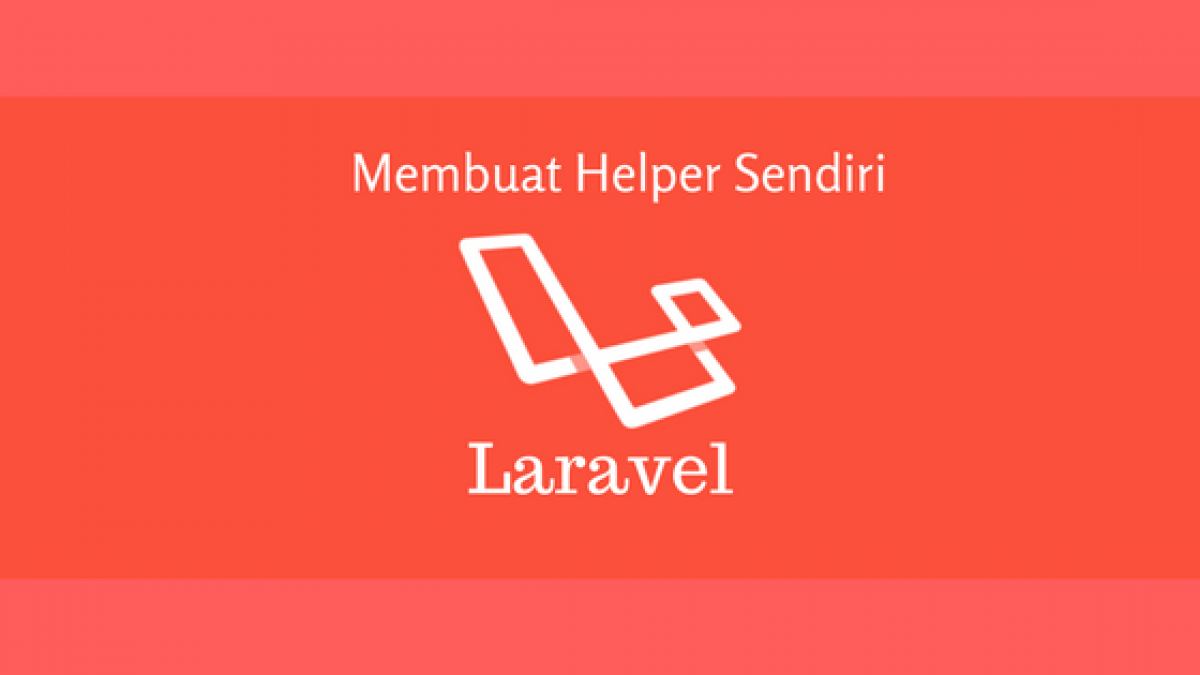
Laravel telah menyediakan beragam helper php function yang bersifat global. Function ini banyak digunakan oleh framework itu sendiri. Namun, anda bebas menggunakannya dalam aplikasi anda jika anda merasa nyaman. Jadi, pada dasarnya, helpers yang disediakan oleh Laravel dapat anda gunakan darimanapun dalam aplikasi anda, jika helpers yang sesuai kebutuhan anda tidak tersedia, maka anda dibolehkan untuk membuat helper sendiri.
Meskipun core Laravel telah menyediakan berbagai macam helper, selalu ada kemungkinan bagi anda untuk membuat helpers anda sendiri dan mengembangkannya sesuai dengan yang anda butuhkan, sehingga anda tidak perlu mengulang kode yang sama berulang kali di berbagai tempat. Hal ini akan menyebabkan proses maintenance menjadi lebih mudah untuk dilakukan. Melalui artikel ini, kita akan belajar membuat helpers sendiri.
Baca Juga: Membuat Fitur Upload Menggunakan Ajax
Membuat Custom Helper
Seperti yang telah kita bahas sebelumnya bahwa Laravel telah menyediakan helpers yang dapat digunakan sesuai kebutuhan kita, helpers tersebut dibagi menjadi beberapa kategori, diantaranya: Arrays, Paths, Strings, URLs, Miscellaneous. Adapun helpers secara rinci dapat anda cek : https://laravel.com/docs/5.5/helpers
Maka terlepas dari helpers yang telah disediakan berdasarkan kegunaannya masing-masing, pada kesempatan kali ini kita akan mencoba untuk membuat custom helper yang dapat digunakan secara global pada aplikasi anda.
Agar mudah dimengerti, maka kita akan membuat helper sederhana dimana kita akan mengambil userid dan mengembalikan username sebagai response. Tampaknya terlalu sederhana, akan tetapi cukup untuk memahami konsepnya sehingga anda dapat mengembangkannya lebih lanjut sesuai kebutuhan anda.
Saya berasumsi anda telah memiliki users table pada database anda dan setidaknya memiliki dua field: userid dan username.
Membuat file helpers
Sebenarnya anda dapat menempatkan file helper yang anda miliki dimanapun pada aplikasi anda. Pada artikel kali ini kita akan bersepakat untuk menempatkannya pada direktori app. Buat folder Helpers dan didalam folder Helpers, buat file User.php kemudian tambahkan potongan code dibawah ini:
<?php
namespace App\Helpers;
use Illuminate\Support\Facades\DB;
class User {
public static function get_username($user_id) {
$user = DB::table('users')->where('userid', $user_id)->first();
return (isset($user->username) ? $user->username : '');
}
}
Langkah selanjutnya adalah dengan membuat service provider, jalankan command berikut:
php artisan make:provider UserServiceProvider
Buka file app/Providers/UserServiceProvider.php
maka anda akan mendapatkan dua buah method. Pada method register, tambahkan potongan code berikut:
public function register()
{
require_once app_path() . '/Helpers/User.php';
}
Kita perlu menginformasikan bahwa kita memiliki service provider baru agar dapat digunakan, buka file config/app.php
dan tambahkan potongan code berikut pada bagian providers
:
App\Providers\UserServiceProvider::class,
Cara mudah menggunakan helper yang kita miliki adalah dengan menambahkan alias, jadi tambahkan code berikut pada bagian aliases
:
'UserHelp' => App\Helpers\User::class,
Dengan menentukan entri ini, maka kita dapat menggunakan helper kita dengan keyword UserHelp
. Adapun code lengkap dari config/app.php
menjadi:
<?php
return [
/*
|--------------------------------------------------------------------------
| Application Name
|--------------------------------------------------------------------------
|
| This value is the name of your application. This value is used when the
| framework needs to place the application's name in a notification or
| any other location as required by the application or its packages.
*/
'name' => env('APP_NAME', 'Laravel'),
/*
|--------------------------------------------------------------------------
| Application Environment
|--------------------------------------------------------------------------
|
| This value determines the "environment" your application is currently
| running in. This may determine how you prefer to configure various
| services your application utilizes. Set this in your ".env" file.
|
*/
'env' => env('APP_ENV', 'production'),
/*
|--------------------------------------------------------------------------
| Application Debug Mode
|--------------------------------------------------------------------------
|
| When your application is in debug mode, detailed error messages with
| stack traces will be shown on every error that occurs within your
| application. If disabled, a simple generic error page is shown.
|
*/
'debug' => env('APP_DEBUG', false),
/*
|--------------------------------------------------------------------------
| Application URL
|--------------------------------------------------------------------------
|
| This URL is used by the console to properly generate URLs when using
| the Artisan command line tool. You should set this to the root of
| your application so that it is used when running Artisan tasks.
|
*/
'url' => env('APP_URL', 'http://localhost'),
/*
|--------------------------------------------------------------------------
| Application Timezone
|--------------------------------------------------------------------------
|
| Here you may specify the default timezone for your application, which
| will be used by the PHP date and date-time functions. We have gone
| ahead and set this to a sensible default for you out of the box.
|
*/
'timezone' => 'UTC',
/*
|--------------------------------------------------------------------------
| Application Locale Configuration
|--------------------------------------------------------------------------
|
| The application locale determines the default locale that will be used
| by the translation service provider. You are free to set this value
| to any of the locales which will be supported by the application.
|
*/
'locale' => 'en',
/*
|--------------------------------------------------------------------------
| Application Fallback Locale
|--------------------------------------------------------------------------
|
| The fallback locale determines the locale to use when the current one
| is not available. You may change the value to correspond to any of
| the language folders that are provided through your application.
|
*/
'fallback_locale' => 'en',
/*
|--------------------------------------------------------------------------
| Encryption Key
|--------------------------------------------------------------------------
|
| This key is used by the Illuminate encrypter service and should be set
| to a random, 32 character string, otherwise these encrypted strings
| will not be safe. Please do this before deploying an application!
|
*/
'key' => env('APP_KEY'),
'cipher' => 'AES-256-CBC',
/*
|--------------------------------------------------------------------------
| Logging Configuration
|--------------------------------------------------------------------------
|
| Here you may configure the log settings for your application. Out of
| the box, Laravel uses the Monolog PHP logging library. This gives
| you a variety of powerful log handlers / formatters to utilize.
|
| Available Settings: "single", "daily", "syslog", "errorlog"
|
*/
'log' => env('APP_LOG', 'single'),
'log_level' => env('APP_LOG_LEVEL', 'debug'),
/*
|--------------------------------------------------------------------------
| Autoloaded Service Providers
|--------------------------------------------------------------------------
|
| The service providers listed here will be automatically loaded on the
| request to your application. Feel free to add your own services to
| this array to grant expanded functionality to your applications.
|
*/
'providers' => [
/*
* Laravel Framework Service Providers...
*/
Illuminate\Auth\AuthServiceProvider::class,
Illuminate\Broadcasting\BroadcastServiceProvider::class,
Illuminate\Bus\BusServiceProvider::class,
Illuminate\Cache\CacheServiceProvider::class,
Illuminate\Foundation\Providers\ConsoleSupportServiceProvider::class,
Illuminate\Cookie\CookieServiceProvider::class,
Illuminate\Database\DatabaseServiceProvider::class,
Illuminate\Encryption\EncryptionServiceProvider::class,
Illuminate\Filesystem\FilesystemServiceProvider::class,
Illuminate\Foundation\Providers\FoundationServiceProvider::class,
Illuminate\Hashing\HashServiceProvider::class,
Illuminate\Mail\MailServiceProvider::class,
Illuminate\Notifications\NotificationServiceProvider::class,
Illuminate\Pagination\PaginationServiceProvider::class,
Illuminate\Pipeline\PipelineServiceProvider::class,
Illuminate\Queue\QueueServiceProvider::class,
Illuminate\Redis\RedisServiceProvider::class,
Illuminate\Auth\Passwords\PasswordResetServiceProvider::class,
Illuminate\Session\SessionServiceProvider::class,
Illuminate\Translation\TranslationServiceProvider::class,
Illuminate\Validation\ValidationServiceProvider::class,
Illuminate\View\ViewServiceProvider::class,
App\Providers\UserServiceProvider::class,
/*
* Package Service Providers...
*/
Laravel\Tinker\TinkerServiceProvider::class,
/*
* Application Service Providers...
*/
App\Providers\AppServiceProvider::class,
App\Providers\AuthServiceProvider::class,
// App\Providers\BroadcastServiceProvider::class,
App\Providers\EventServiceProvider::class,
App\Providers\RouteServiceProvider::class,
],
/*
|--------------------------------------------------------------------------
| Class Aliases
|--------------------------------------------------------------------------
|
| This array of class aliases will be registered when this application
| is started. However, feel free to register as many as you wish as
| the aliases are "lazy" loaded so they don't hinder performance.
|
*/
'aliases' => [
'App' => Illuminate\Support\Facades\App::class,
'Artisan' => Illuminate\Support\Facades\Artisan::class,
'Auth' => Illuminate\Support\Facades\Auth::class,
'Blade' => Illuminate\Support\Facades\Blade::class,
'Broadcast' => Illuminate\Support\Facades\Broadcast::class,
'Bus' => Illuminate\Support\Facades\Bus::class,
'Cache' => Illuminate\Support\Facades\Cache::class,
'Config' => Illuminate\Support\Facades\Config::class,
'Cookie' => Illuminate\Support\Facades\Cookie::class,
'Crypt' => Illuminate\Support\Facades\Crypt::class,
'DB' => Illuminate\Support\Facades\DB::class,
'Eloquent' => Illuminate\Database\Eloquent\Model::class,
'Event' => Illuminate\Support\Facades\Event::class,
'File' => Illuminate\Support\Facades\File::class,
'Gate' => Illuminate\Support\Facades\Gate::class,
'Hash' => Illuminate\Support\Facades\Hash::class,
'Lang' => Illuminate\Support\Facades\Lang::class,
'Log' => Illuminate\Support\Facades\Log::class,
'Mail' => Illuminate\Support\Facades\Mail::class,
'Notification' => Illuminate\Support\Facades\Notification::class,
'Password' => Illuminate\Support\Facades\Password::class,
'Queue' => Illuminate\Support\Facades\Queue::class,
'Redirect' => Illuminate\Support\Facades\Redirect::class,
'Redis' => Illuminate\Support\Facades\Redis::class,
'Request' => Illuminate\Support\Facades\Request::class,
'Response' => Illuminate\Support\Facades\Response::class,
'Route' => Illuminate\Support\Facades\Route::class,
'Schema' => Illuminate\Support\Facades\Schema::class,
'Session' => Illuminate\Support\Facades\Session::class,
'Storage' => Illuminate\Support\Facades\Storage::class,
'URL' => Illuminate\Support\Facades\URL::class,
'Validator' => Illuminate\Support\Facades\Validator::class,
'View' => Illuminate\Support\Facades\View::class,
'UserHelp' => App\Helpers\User::class,
],
];
Baca Juga: Kumpulan Ikon Terbaik Untuk Desain Web
#### Menggunakan Custom HelperHelper yang kita inginkan telah berhasil dibuat, langkah selanjutnya adalah kita mencoba menggunakan helper ini. Agar tampak mudah dan dapat dipahami maka kita akan menggunakan helper ini secara langsung menggunakan closure method. Buka file routes/web.php
kemudian tambahkan potongan code berikut:
Route::get('/helper-user', function () {
return UserHelp::get_username(1);
});
Maka ketika anda mengakses url /helper-user
, anda akan mendapatkan username dengan userid = 1. Anda dapat menggunakan custom helper darimanapun anda inginkan, seperti: dari controller atau views.